Operator, if, for loop 코드 리뷰
이번 시간에는 엘리님 강의의 4번째인 Operator, if, for loop 코드 리뷰 라는 제목의 강의를 공부 해 보자.
즉 한국말로는 연산 명령어들이다.
1. 문자열 병합
즉 문자와 문자를 병합하는 함수를 출력 해 보자.
문자는 따옴표로 속성을 부여 하였고 나중 " console.log " 함수를 사용하여 출력시 결과값 앞에 출력되는 문구를 표시하고 싶을때에는 백틱 " ` " 을 사용한다.
그리고 결과값은 " ${ } " 이 부분이다.
console.log('my'+'cat'); console.log('1'+2); console.log(`string literals: 1 + 2 = ${1 + 2}`);
위에서 첫번째 결과는 쉽게 예상할 수 있겠고 특이한 것은 두번째 결과인데 2 또한 자바스크립트에서는 문자로 간주하므로 " 12 " 가 출력된다.
세번째는 string literlas: 1 + 2 = 까지는 그대로 출력되는데 " ${ } " 안에 1 과 2 모두 숫자로 속성을 부여 하였으므로 3 이 앞에 출력되는 문자 그 다음에 같이 출력된다.
2. 숫자형 연산자
자 이번에는 숫자형 연산자들이다.
첫번째에서 4번째까지는 컴퓨터에서도 일반적으로 많이 행하고 있는 연산자들이므로 익숙할 것이다.
5 번째는 나머지를 구하는 연산자이다.
즉 5 를 2 로 나누면 2 가 되고 나머지가 1 이므로 결과값은 1 이다.
6 번째는 제곱을 나타내는 연산자로서 2 의 3 제곱 이므로 결과값은 8 이다.
console.log(1 + 1); // add console.log(1 - 1); // subtract console.log(1 / 1); // divide console.log(1 * 1); // multiply console.log(5 % 2); // remainder console.log(2 ** 3); // exponentiation
3. Increment and decrement operators
이번에는 각각 명령어 옆에 주석 처리한 것을 직접 보여주면서 설명을 대신하도록 하겠다.
let counter = 2; // 2 를 변수인 counter 에 대입한다. const preIncrement = ++counter; // counter + 1 이므로 3 이 된다. console.log(`preIncrement: ${preIncrement}, counter: ${counter}`); // counter 에 1 을 더한 후 preIncrement 에 넣어줘라고 했으니 preIncrement 와 counter 는 동일하게 3 을 출력한다. const postIncrement = counter++; // ++ 가 뒤에 나왔다. 즉 postIncrement 에 위의 counter 값 3 을 대입하고 그 후에 counter 에 1 을 더한다. console.log(`postIncrement: ${postIncrement}, counter: ${counter}`); // 위에서 counter 는 3 이 되었으니 postIncrement 는 3 이 되고 그리고 나서 counter 에 1을 더하여야 하니 counter 는 4 가 된다. const preDecrement = --counter; console.log(`preDecrement: ${preDecrement}, counter: ${counter}`); // 위에서 counter 는 4 가 되었고 1을 먼저 빼주라고 했으니 preDecrement 는 3 이 되고 counter 또한 3 이 된다. const postDecrement = counter--; console.log(`postDecrement: ${postDecrement}, counter: ${counter}`); // 위에서 counter 는 3 이 되었으니 postDecrement 는 3 이 되고 counter 는 1 을 빼줘야 하니 2 가 된다.
4. Assignment operators
let x = 3; let y = 6; x += y; // x = x + y x -= y; x *= y; x /= y;
5. Comparison operators
console.log(10 < 6); // less than console.log(10 <= 6); // less than or equal console.log(10 > 6); // greater than console.log(10 >= 6); // greater than or equal
6. Logial operators
|| (or), && (and), ! (not) const value1 = false; const value2 = 4 < 2; // || (or) 만일 위에서 value1 을 true 로 지정한다면 아래 함수에서 3가지 값들을 비교할 의미가 없기에 아래 놀라는 마크는 출력하지 않고 바로 true 만 출력이 된다. // 코딩시 팁이라면 아래 비교 함수 작성시 비교 변수 순서를 단순 상수부터 해야지 만일 check 라는 변수부터 진행을 하면 시간이 오래 걸림 console.log(`or: ${value1 || value2 || check()}`); function check() { for (let i = 0; i < 10; i++) { // i 는 0 부터 시작해서 9 까지 총 10 개 값이 true 이므로 놀라는 이모지가 10 번 출력된다. // wasting time console.log('😱'); } return true; } // && (and) const value3 = true; const value4 = 4 > 2; console.log(`and: ${value3 && value4 && check()}`); function check() { for (let i = 0; i < 10; i++) { // wasting time console.log('😱'); } return true; }
Categories
JavaScript
- Intro ...
- Operator, if, for loop 코드 리뷰
- Operator, if, for loop 코드 리뷰 (2)
- Functions 함수
- 클래스와 오브젝트의 차이점 및 객체지향 언어 클래스
- 버튼 클릭시 배경화면이 다크모드로 전환되게 만들기
- 버튼 클릭시 배경화면이 다크모드로 전환되게 만들기 (2)
- 버튼 클릭시 경고창 띄우기
- 리팩토링
- 자바스크립트 - 계산기
Wizzen2801
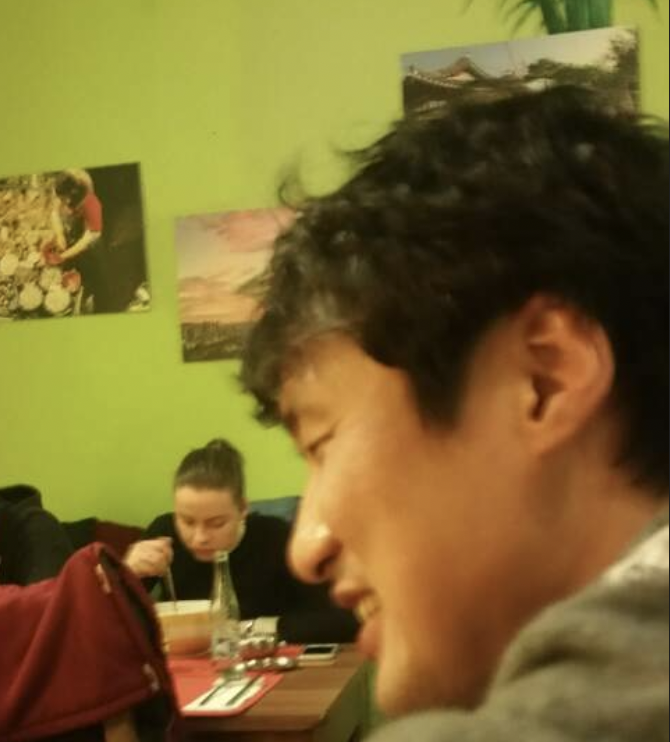